해당 포스트는 R에서 tidyverse 패키지를 이용하여 데이터 필터링하는 방법을 코드와 함께 소개하는 글입니다.
1. INTRO
데이터 프레임(Data Frame)은 R에서 가장 많이 사용하는 자료 구조입니다. 분석을 위해 데이터를 추출하거나 필터링하는 경우, 내장 함수만으로도 처리 가능하지만 tidyverse 패키지(정확히는 tidyverse 패키지에 포함된 dplyr 패키지)를 이용하면 좀 더 직관적이고 효과적인 처리가 가능해 집니다.
R 내장 데이터셋인 msleep
을 이용해 코드와 함께 설명하겠습니다.
패키지 불러오기
library(tidyverse)
head(msleep)
# A tibble: 6 x 11 name genus vore order conservation sleep_total sleep_rem sleep_cycle awake brainwt bodywt1 Cheetah Acinonyx carni Carnivora lc 12.1 NA NA 11.9 NA 50 2 Owl monkey Aotus omni Primates NA 17 1.8 NA 7 0.0155 0.48 3 Mountain beaver Aplodontia herbi Rodentia nt 14.4 2.4 NA 9.6 NA 1.35 4 Greater short-tailed shrew Blarina omni Soricomorpha lc 14.9 2.3 0.133 9.1 0.00029 0.019 5 Cow Bos herbi Artiodactyla domesticated 4 0.7 0.667 20 0.423 600 6 Three-toed sloth Bradypus herbi Pilosa NA 14.4 2.2 0.767 9.6 NA 3.85
2. 데이터 필터링 방법 10가지
Example 1
msleep
데이터에서name
,sleep_total
컬럼 추출sleep_total
값이 15 이상인 행 출력
R코드 및 출력 결과
data1 <- msleep %>%
select(name, sleep_total) %>%
filter(sleep_total > 15)
data1
# A tibble: 12 x 2 name sleep_total1 Owl monkey 17 2 Long-nosed armadillo 17.4 3 North American Opossum 18 4 Big brown bat 19.7 5 Thick-tailed opposum 19.4 6 Little brown bat 19.9 7 Tiger 15.8 8 Giant armadillo 18.1 9 Arctic ground squirrel 16.6 10 Golden-mantled ground squirrel 15.9 11 Eastern american chipmunk 15.8 12 Tenrec 15.6
Example 2
msleep
데이터에서name
,sleep_total
컬럼 추출sleep_total
값이 15 이상인 아닌 행 출력
R코드 및 출력 결과
data2 <- msleep %>%
select(name, sleep_total) %>%
filter(!sleep_total > 15)
data2
# A tibble: 71 x 2 name sleep_total1 Cheetah 12.1 2 Mountain beaver 14.4 3 Greater short-tailed shrew 14.9 4 Cow 4 5 Three-toed sloth 14.4 6 Northern fur seal 8.7 7 Vesper mouse 7 8 Dog 10.1 9 Roe deer 3 10 Goat 5.3 # ... with 61 more rows
Example 3
msleep
데이터에서name
,order
,bodywt
,sleep_total
컬럼 추출order
값이 'Primates' 이고,bodywt
값이 15를 초과하는 행 출력
R코드 및 출력 결과
data3 <- msleep %>%
select(name, order, bodywt, sleep_total) %>%
filter(order == "Primates", bodywt > 15)
data3
# A tibble: 3 x 4 name order bodywt sleep_total1 Human Primates 62 8 2 Chimpanzee Primates 52.2 9.7 3 Baboon Primates 25.2 9.4
Example 4
msleep
데이터에서name
,order
,bodywt
,sleep_total
컬럼 추출order
값이 'Primates' 이거나,bodywt
값이 15를 초과하는 행 출력
R코드 및 출력 결과
data4 <- msleep %>%
select(name, order, bodywt, sleep_total) %>%
filter(order == "Primates" | bodywt > 15)
data4
# A tibble: 33 x 4 name order bodywt sleep_total1 Cheetah Carnivora 50 12.1 2 Owl monkey Primates 0.48 17 3 Cow Artiodactyla 600 4 4 Northern fur seal Carnivora 20.5 8.7 5 Goat Artiodactyla 33.5 5.3 6 Grivet Primates 4.75 10 7 Asian elephant Proboscidea 2547 3.9 8 Horse Perissodactyla 521 2.9 9 Donkey Perissodactyla 187 3.1 10 Patas monkey Primates 10 10.9 # ... with 23 more rows
Example 5
msleep
데이터에서name
,sleep_total
컬럼 추출name
값이 'Cow' 또는 'Dog' 또는 'Goat' 인 행 출력
R코드 및 출력 결과
data5 <- msleep %>%
select(name, sleep_total) %>%
filter(name == "Cow" |
name == "Dog" |
name == "Goat")
data5
# A tibble: 3 x 2 name sleep_total1 Cow 4 2 Dog 10.1 3 Goat 5.3
Example 6
msleep
데이터에서name
,sleep_total
컬럼 추출name
값 중에 c('Cow','Dog','Goat') 벡터에 속하는 행 추출 (Example 5와 같은 의미)
R코드 및 출력 결과
data6 <- msleep %>%
select(name, sleep_total) %>%
filter(name %in% c("Cow", "Dog", "Goat"))
data6
# A tibble: 3 x 2 name sleep_total1 Cow 4 2 Dog 10.1 3 Goat 5.3
Example 7
msleep
데이터에서name
,sleep_total
컬럼 추출sleep_total
값이 16 에서 18 사이인 행 추출
R코드 및 출력 결과
data7 <- msleep %>%
select(name, sleep_total) %>%
filter(between(sleep_total, 16, 18))
data7
# A tibble: 4 x 2 name sleep_total1 Owl monkey 17 2 Long-nosed armadillo 17.4 3 North American Opossum 18 4 Arctic ground squirrel 16.6
Example 8
msleep
데이터에서name
,sleep_total
컬럼 추출sleep_total
값이 17 근처인 행 추출 (편차 = 0.5)
R코드 및 출력 결과
data8 <- msleep %>%
select(name, sleep_total) %>%
filter(near(sleep_total, 17, tol = 0.5))
data8
# A tibble: 3 x 2 name sleep_total1 Owl monkey 17 2 Long-nosed armadillo 17.4 3 Arctic ground squirrel 16.6
Example 9
msleep
데이터에서name
,conservation
,sleep_total
컬럼 추출conservation
값이NA
인 행 추출
R코드 및 출력 결과
data9 <- msleep %>%
select(name, conservation, sleep_total) %>%
filter(is.na(conservation))
data9
# A tibble: 29 x 3 name conservation sleep_total1 "Owl monkey" NA 17 2 "Three-toed sloth" NA 14.4 3 "Vesper mouse" NA 7 4 "African giant pouched rat" NA 8.3 5 "Western american chipmunk" NA 14.9 6 "Galago" NA 9.8 7 "Human" NA 8 8 "Macaque" NA 10.1 9 "Vole " NA 12.8 10 "Little brown bat" NA 19.9 # ... with 19 more rows
Example 10
msleep
데이터에서name
,conservation
,sleep_total
컬럼 추출conservation
값이NA
가 아닌 행 추출
R코드 및 출력 결과
data10 <- msleep %>%
select(name, conservation, sleep_total) %>%
filter(!is.na(conservation))
data10
# A tibble: 54 x 3 name conservation sleep_total1 Cheetah lc 12.1 2 Mountain beaver nt 14.4 3 Greater short-tailed shrew lc 14.9 4 Cow domesticated 4 5 Northern fur seal vu 8.7 6 Dog domesticated 10.1 7 Roe deer lc 3 8 Goat lc 5.3 9 Guinea pig domesticated 9.4 10 Grivet lc 10 # ... with 44 more rows
3. 관련 링크
[1] [R-bloggers] Filtering Data in R 10 Tips -tidyverse package
[2] [RDocumentation] dplr::near - Compare two numeric vectors
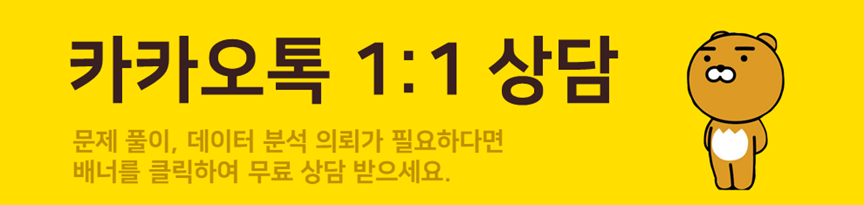