해당 포스트는 Hadley Wickham이 작성한 'The tidyverse style guide' 를 번역하여 정리한 글입니다.
Lists
Intro - 0. Welcome
Analysis - 1. Files
Analysis - 2. Syntax (1)
Analysis - 2. Syntax (2)
Analysis - 3. Functions
Analysis - 4. Pipes
Analysis - 5. ggplot2
Packages - 6. Files
Packages - 7. Documentation
Packages - 8. Tests
Packages - 9. Error messages
Packages - 10. News
Packages - 11. Git/GitHub
3. 함수(Functions)
3.1 함수 이름 생성(Naming)
객체 이름(Object Names)에 대한 일반적인 조언(규칙)을 따르는 것 외에도 함수 이름에 동사를 사용하도록 노력하는 것이 좋습니다.
# Good
add_row()
permute()
# Bad
row_adder()
permutation()
3.2 긴 코드 처리(Long Lines)
만약 함수를 정의하는 코드가 여러 줄로 작성된다면, 함수 정의가 시작되는 위치로 두 번째 줄을 들여쓰는(indent) 것이 좋습니다.
# Good
long_function_name <- function(a = "a long argument",
b = "another argument",
c = "another long argument") {
# As usual code is indented by two spaces.
}
# Bad
long_function_name <- function(a = "a long argument",
b = "another argument",
c = "another long argument") {
# Here it's hard to spot where the definition ends and the
# code begins
}
3.3 함수 결과 반환(return)
return()
명령어는 함수 결과의 조기 반환(return)이 필요할 때에만 사용합니다. 보통의 경우, 마지막으로 실행된 표현식(expression)의 결과를 반환합니다.
# Good
find_abs <- function(x) {
if (x > 0) {
return(x)
}
x * -1
} # return(x)의 결과 출력
add_two <- function(x, y) {
x + y
} # x + y 의 결과 출력
# Bad
add_two <- function(x, y) {
return(x + y)
}
리턴(return) 문은 제어 흐름에 중요한 영향을 미치므로 항상 자체 라인(독립된 라인)에 있어야 합니다.
# Good
find_abs <- function(x) {
if (x > 0) {
return(x)
}
x * -1
}
# Bad
find_abs <- function(x) {
if (x > 0) return(x)
x * -1
}
만약 작성한 함수가 print()
, plot()
, save()
등의 외부 작용으로 호출된다면, 첫 번째 인자(argument)를 보이지 않게 반환(return)해야 합니다. 이는 파이프(pipe)의 일부로 사용하는 것을 가능하게 합니다.(여러 함수들을 파이프(pipe)와 같이 연결해서 사용해야 할 때 필요한 인자만 출력할 수 있도록 도와줍니다.)print
메서드는 대부분 아래 httr의 예와 같이 사용해야 합니다.
print.url <- function(x, ...) {
cat("Url: ", build_url(x), "\n", sep = "")
invisible(x)
}
Function : invisible()
기본적으로 R에서는 함수 실행 결과를 출력하게 되어 있습니다.invisible()
함수를 사용하면 출력여부를 설정할 수 있으며, 이는 파이프(pipe)의 부분 함수로 사용하는데 도움을 줍니다.
3.4 주석(Comments)
코드의 주석(Comments)의 목적은 '무엇(What)' 또는 '어떻게(How)'가 아닌 '왜(Why)'를 설명하는 것입니다. 주석의 각 줄은 주석 기호(#
)와 단일 공백()으로 시작해야 합니다.
# Good
# Objects like data frames are treated as leaves
x <- map_if(x, is_bare_list, recurse)
# Bad
# Recurse only with bare lists
x <- map_if(x, is_bare_list, recurse)
주석은 문장으로 구성되어야 하며, 적어도 2개 이상의 문장을 포함하는 경우에만 마침표(.
)로 끝내야 합니다.
# Good
# Objects like data frames are treated as leaves
x <- map_if(x, is_bare_list, recurse)
# Do not use `is.list()`. Objects like data frames must be treated
# as leaves.
x <- map_if(x, is_bare_list, recurse)
# Bad
# objects like data frames are treated as leaves
x <- map_if(x, is_bare_list, recurse)
# Objects like data frames are treated as leaves.
x <- map_if(x, is_bare_list, recurse)
)
출처
[1] The tidyverse style guide
[2] 김용환님 블로그 : [R] invisible()
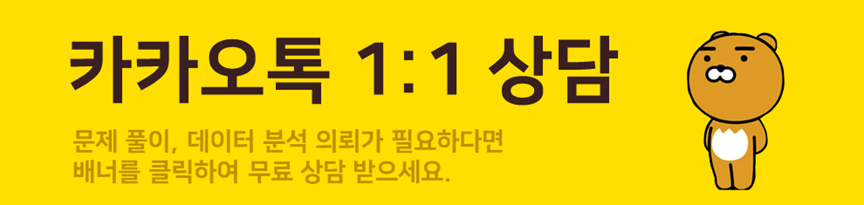